1. 明确功能
目标
程序开始运行时显示一个迷宫地图,给定入口和出口,游戏的任务是使用键盘上的方向键操纵老鼠在规定的时间内走到出口处。
内容
给定入口和出口,随机生成一个可以走出去的迷宫。老鼠从入口位置出发,要求求出从迷宫入口到出口有无通路,若有通路则指出其中一条通路的路径,即输出找到通路的迷宫数组,其中通路上的“0”用另外一个数字8替换,同时打印出所走通路径上每一步的位置坐标及下一步的方向。
要求
- 老鼠形象可辨认,可用键盘操纵老鼠上下左右移动;
- 迷宫的墙足够结实,老鼠不能穿墙而过;
- 正确检测结果,若老鼠在规定时间内走到出口处,提示成功,否则提示失败;
- 添加编辑迷宫功能,可修改当前迷宫,修改内容:墙变路、路变墙;
- 找出走出迷宫的所有路径,以及最短路径。
利用序列化功能实现迷宫地图文件的存盘和读出等功能
2. 实现过程
1)整个地图使用二维字符实现
2)流程图
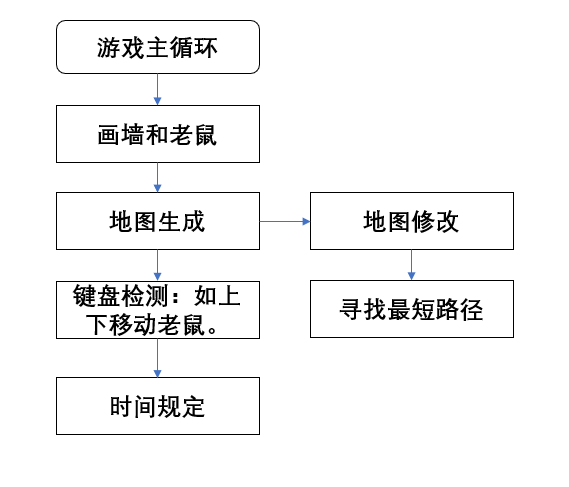
3.代码
1) 老鼠走迷宫的类:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| class mouse_maze{ public: void initialize(); int rand_int(int a, int b); void gameshow(); void gamemazeshow(); int run(); void storage(); void reading(); void change(int n,int m); void gametime(); int judge(int i,int j); public: char s[N][N]; int success=0; int head=0; char key; int x=1,y=0; int x1,y1; };
|
2) 初始化随机生成一个走得出去的迷宫
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| void mouse_maze::initialize() { int i, j; int flag=0; for (int i=0;i<N;i++) { s[0][i]='$'; s[N-1][i]='$'; } for(int j=1;j<N-1;j++) { if(j==1) { s[j][0]='*'; s[j][N-1]='$'; } else if(j==N-2) { s[j][0]='$'; s[j][N-1]='8'; } else { s[j][0]='$'; s[j][N-1]='$'; } } for(int i=1;i<N-1;i++) for(int j=1;j<N-1;j++) s[i][j]=rand_int(0,1)+'0'; while(judge(1,1)==0) { for(int i=1;i<N-1;i++) for(int j=1;j<N-1;j++) s[i][j]=rand_int(0,1)+'0'; } }
|
3)判断从迷宫入口到出口有无通路
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| int mouse_maze::judge(int i,int j) { s[i][j]='8'; if(i==N-2&&j==N-2) success=1; if(success!=1&&s[i][j+1]=='0') judge(i,j+1); if(success!=1&&s[i+1][j]=='0') judge(i+1,j); if(success!=1&&s[i][j-1]=='0') judge(i,j-1); if(success!=1&&s[i-1][j]=='0') judge(i-1,j);
if(success!=1) s[i][j]='0'; return success; }
|
4) 显示迷宫的全部内容
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| void mouse_maze::gameshow() { system("cls"); int i, j; cout<<endl; for(int i=0;i<N;i++) { cout<<"\t"; for(int j=0;j<N;j++) cout<<s[i][j]; cout<<endl; } }
|
5) 显示进行游戏时的迷宫内容
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| void mouse_maze::gamemazeshow() { int i, j; cout<<endl; for(int i=0;i<N;i++) { cout<<"\t"; for(int j=0;j<N;j++) { if(s[i][j]=='1'||s[i][j]=='$') cout<<"$"; else if(s[i][j]=='*') cout<<"*"; else cout<<" "; } if(i==0) { cout<<"\t\t游戏计时:"; } cout<<endl; } cout<<endl<<"注:按w向上移动,按s向下移动,按a向左移动,按d向右移动"<<endl; }
|
6) 将迷宫存储到文件中和从文件读取迷宫
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| void mouse_maze::reading() { ifstream read("maze.txt",ios::in); if(!read) { cout<<"family.txt文件不存在"; system("pause"); exit(0); } for(int i=0;i<N;i++) for(int j=0;j<N;j++) read>>s[i][j]; } void mouse_maze::storage() { ofstream output("maze.txt", ios::out); for(int i=0;i<N;i++) { for(int j=0;j<N;j++) output<<s[i][j]<<" "; output<<endl; } }
|
7) 改变迷宫
1 2 3 4 5 6 7
| void mouse_maze::change(int n,int m) { if(s[n-1][m-1]=='1') s[n-1][m-1]='0'; else if(s[n-1][m-1]=='0'||s[n-1][m-1]=='8') s[n-1][m-1]='1'; }
|
8) 游戏运行
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| int mouse_maze::run() { int flag=0; switch(getch()) { case 119:x1=x-1;y1=y ;break; case 115:x1=x+1;y1=y ;break; case 97:x1=x;y1=y-1 ;break; case 100:x1=x;y1=y+1 ;break; default:cout<<"重新输入:" ;break; } if(s[N-2][N-1]=='*') { flag=1; } else if(s[x1][y1]=='0'||s[x1][y1]=='8') { s[x][y]='0'; s[x1][y1]='*'; gamemazeshow(); x=x1; y=y1; } else { gamemazeshow(); getch(); } return flag; }
|